Foreign Function Interface (FFI) for Enhanced Flutter App Development
FFI also known as Foreign Function Interface (FFI) allows us to call functions written in a different programming language like C, C++, or Rust, directly from our Flutter Dart code. This is particularly helpful when adding platform-specific libraries or performing low-level tasks. In this article, we will discuss Flutter FFI Integration for Improved App Development.
How Flutter FFI Works
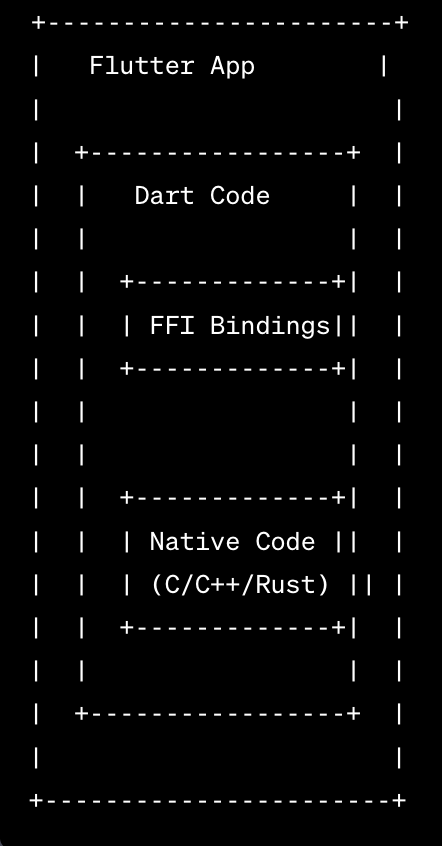
You will define FFI bindings, also known as Dart FFI bindings that specify how your Dart code will interact with native functions and data types. These bindings serve as a contract between your code and native libraries and define which functions can be called and how data should be passed between them.
To help interact with native functions, FFI provides DynamicLibrary.open
and lookupFunction
. In your Dart code, import dart:ffi library. You will see examples of how to use these functions in the sections below.
Benefits of Flutter FFI
Flutter FFI integration helps us bridge the gap between our Flutter application and native code. This allows us to:
1. Access Native Libraries
You can use Flutter FFI to access native libraries written in C, C++, or Rust. This allows you to interface with platform-specific APIs, hardware sensors, or multimedia codecs. Let’s see an example:
In this example, consider we have a native C library (calculate_square_example
) with a function calculateSquare
that we want to call from our Flutter app.
// calculate_square_example.c
#include <stdio.h>
int calculateSquare(int number) {
return number * number;
}
Now let’s create Flutter FFI binding to access calculateSquare
function.
// ffi_bindings.dart
import 'dart:ffi'; // Import the Dart FFI library
final DynamicLibrary myNativeLibrary = DynamicLibrary.open('calculate_square_example.so');
typedef CalculateSquareC = Int32 Function(Int32);
typedef CalculateSquareDart = int Function(int);
final calculateSquare = myNativeLibrary
.lookupFunction<CalculateSquareC, CalculateSquareDart>('calculateSquare');
2. Performance Optimization
You can use Flutter FFI to perform high CPU or memory-oriented tasks in native code, which results in better performance compared to doing the same task in pure Dart code. Since the native libraries are usually highly optimized, this helps in a better-performing application.
3. Memory Management
Flutter FFI provides tools like calloc, malloc, free
, etc. to handle memory allocation and deallocation. This results in you having more control over the memory management of the application. Here’s an example:
import 'dart:ffi';
final DynamicLibrary myLibrary = DynamicLibrary.open('my_library.so');
// Allocate memory for an int
final intPointer = calloc<Int32>();
// Call a native function that uses the intPointer
myLibrary.callNativeFunction(intPointer);
// Free allocated memory when done
calloc.free(intPointer);
4. Reusing existing code/libraries
As demonstrated in the above examples, using FFI, you can add your existing native libraries and code to the Flutter app. This helps in removing the need of porting the native libraries into Dart code and thus saves time and effort.
5. Custom Memory Management
Depending on your needs, you can use Dart and Flutter FFI to create custom memory management strategies. This involves managing memory pools, reference counting, and other such techniques.
Accessing Native Android/iOS code
If you want to access your native Android or iOS code instead of some C, C++ or Rust code, you can use the Event Channel or Method channel.
Considerations
While FFI is a great tool, there are some important considerations to keep in mind when working with it. Please be mindful of the memory management and resource cleanup after using a native function. Also, test the application thoroughly to ensure that all the edge cases are handled and any exception or error thrown by the native code is gracefully handled. Although using native code generally brings performance improvements, sometimes the overhead of using FFI outweighs the performance gains.
By testing and measuring the app performance thoroughly, you can ensure that the FFI integration not only adds native functionality to your application but does not harm it. It’s a great tool but remember, with great power, comes greater responsibility. Flutter docs have provided excellent documentation on how to use FFI on Android or iOS. There is also an excellent codelab on using FFI in a Flutter plugin.